Software Engineering Fundamentals - Design Patterns
- Biyi Akinpelu
- Jan 28, 2023
- 3 min read
Updated: Feb 3, 2023
Design patterns are a set of solutions to common problems that software engineers encounter when building software. These patterns are not specific to any particular programming language or framework, but rather are general solutions that can be applied to a wide range of software development challenges. A Design Pattern is a general, reusable solution to a commonly recurring problem within a given context in software design.
There are several types of design patterns, including Creational patterns, Structural patterns, and Behavioral patterns. Design Pattern Examples
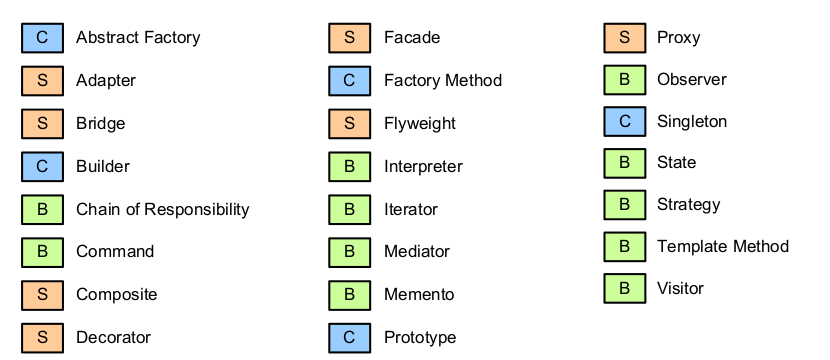
Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Structural patterns deal with object composition, creating relationships between objects to form larger structures. Behavioral patterns focus on communication between objects.
One of the most widely used Creational patterns is the Factory pattern. This pattern is used to create objects without specifying the exact class of object that will be created. The factory pattern uses a factory class to create objects based on a set of input parameters. This allows for a level of flexibility in the code, as the factory class can be easily modified to create different types of objects depending on the needs of the application.
Another common creational pattern is the Singleton pattern, which ensures that a class has only one instance and provides a global point of access to it. Singleton pattern is often used in situations where an application needs to maintain a single connection to a database or a single instance of a logging class, for example.
Structural patterns include the Adapter pattern, which allows incompatible classes to work together by wrapping an interface around one class and adapting it to the interface of another class. The Decorator pattern, which allows new behavior to be added to an existing object without modifying its class. This pattern is often used in situations where an application needs to add new functionality to an existing class without making changes to the underlying code.
Behavioral patterns include the Observer pattern, which allows objects to be notified of changes to other objects, and the Iterator pattern, which allows objects to be iterated over in a consistent manner.
It is important to note that design patterns are not a one-size-fits-all solution. They are meant to be used as a guide, not as a strict set of rules. The appropriate use of design patterns will depend on the specific requirements of the software being developed and the skills of the developers working on the project.
Design patterns can be a valuable tool for software engineers, helping them to write more maintainable and flexible code. By understanding and applying these patterns, developers can create software that is more easily extended and adapted to changing requirements. It is therefore recommended that software engineers make themselves familiar with design patterns and incorporate them into their development process as appropriate. Design Patterns Summary
Design Patterns are reusable solutions to common software development problems, aimed at improving design quality and code maintainability.
To summarize all knowledge learned from Refactoring Guru on Design Patterns. Download the Design Pattern Cheatsheet
You can download this cheatsheet as an additional resource.
Recommended Book on Design Patterns
Design Patterns: Elements of Reusable Object-Oriented Software
Design Patterns: Elements of Reusable Object-Oriented Software is a book that describes common patterns used in object-oriented software design. It was written by Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides, known as the Gang of Four (GOF). The book presents 23 design patterns that can be used to solve common software development problems in a reusable, elegant, and efficient way. It is considered as a classic reference in the field of software engineering.
Have a look at my code examples for more on Design Patterns on GitHub. Also, read through this coding best practices to help you with improving the quality of your code.
Comments